Stepping through a Ray Trace
The following script is provided as an example of stepping a single ray through a ray trace to extract the ray path length and intersection list, much as one might do with the Single Ray Trace tool from the User Interface.
'#Language "WWB-COM" Option Explicit 'Script to step through a trace one intersection at a time and extract the ray path length and the surface intersected 'Note this method assumes that only one source is active with a single ray defined and no ray splitting occurs during the trace. Sub Main Dim adv As T_ADVANCEDRAYTRACE, ray As T_RAY, sNode As Long, continue As Boolean, n As Long 'Set up advanced trace to step through the surfaces one intersection at a time InitAdvancedRaytrace(adv) adv.hitCount = 1 adv.traceActiveSources = False CreateSources GetRay(n, ray) 'get the ray (there is only one) sNode = ray.entity 'get the starting ray entity Print "" Print "Pathlength" & Chr(9) & "Node Name" 'print some headers Print ray.pathlength & Chr(9) & GetFullName(ray.entity) ' print starting ray data continue = True 'set condition to enter the loop While continue EnableTextPrinting(False) AdvancedRaytrace(adv) ' perform the trace EnableTextPrinting(True) GetRay(n, ray) ' get the new ray If ray.entity <> sNode Then 'test ray has moved to a new surface - if so then print information needed Print ray.pathlength & Chr(9) & GetFullName(ray.entity) sNode = ray.entity Else ' ran out of intersections - exit loop continue = False End If Wend End Sub
To test this script we could run it on the tutorialSLRBasic.frd file found in the folder:
\Resources\Samples\Tutorials & Examples
Making sure to change the source definition to be a 1x1 ray grid (i.e. containing only one ray). This system is already set up such that no ray splitting occurs.
The result of executing the script is found in the output window as follows:
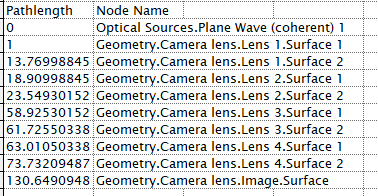